Initializing the SDK
Overall Flow
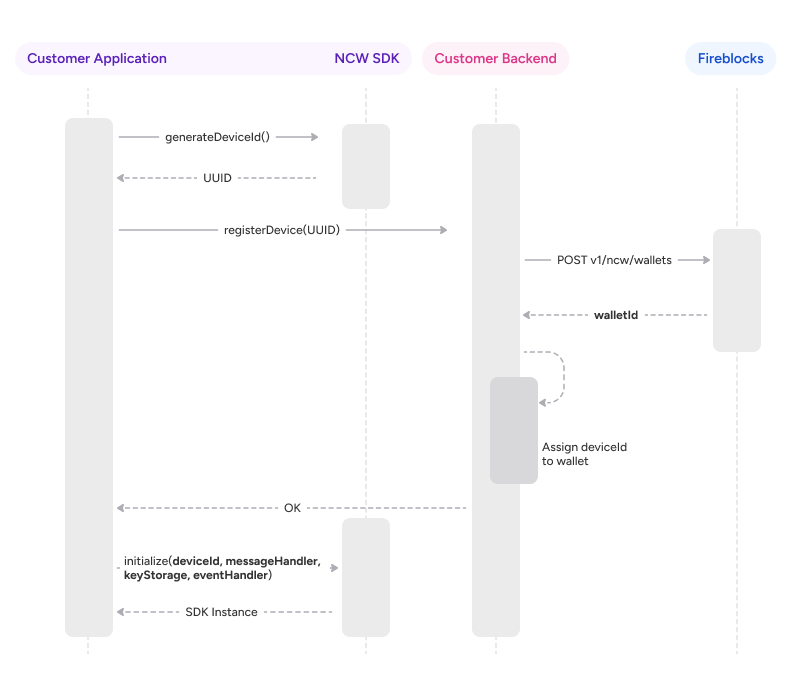
- Generate a device ID by calling the
generateDeviceId()
static method of the SDK. - Assign the
deviceId
to a wallet on your backend server. (POST /v1/wallets/
can be executed at this point.) - Initialize a new SDK instance.
Initialize the SDK
Generate a deviceId
. This value is unique per SDK instance.
Each non-custodial wallet (NCW) walletId
correlates to a single deviceId
:
// generate a device Id, do it only once per SDK instance
const deviceId = FireblocksNCW.generateDeviceId()
// generate a device ID, do it only once per SDK instance
val deviceId = Fireblocks.generateDeviceId();
// generate a device Id, do it only once per SDK instance
let deviceId = Fireblocks.generateDeviceId()
Create an SDK instance
When creating a new SDK instance, provide the NCW instance with the following parameters:
deviceId
: the device ID associated with the end user and the Non Custodial WalletmessagesHandler
: the outgoing message handlerkeyStorage
: the key storage handlereventsHandler
(optional): the events handler
Example
import { FireblocksNCW, IEventsHandler, IMessagesHandler, TEvent, InMemorySecureStorageProvider } from "@fireblocks/ncw-js-sdk";
// Initiate secure storage to hold generated data during SDK usage.
const secureStorageProvider = new InMemorySecureStorageProvider();
// Register a message handler to process outgoing message to your API
const messagesHandler: IMessagesHandler = {
handleOutgoingMessage: (message: string) => {
// Send the message to your API service
},
};
// Register an events handler to handle on various events that the SDK emitts
const eventsHandler: IEventsHandler = {
handleEvent: (event: TEvent) => {
if (event.type === 'key_descriptor_changed') {
// Do something when the event is fired.
}
},
};
// Initialize Fireblocks NCW SDK
const fireblocksNCW = await FireblocksNCW.initialize(
deviceId,
messagesHandler,
eventsHandler,
secureStorageProvider,
);
// create your own implementation of the FireblocksMessageHandler
val fireblocksMessageHandler = FireblocksMessageHandlerImpl()
// create your own implementation of the FireblocksKeyStorage
val fireblocksKeyStorage = FireblocksKeyStorageImpl()
// build your FireblocksOptions instance
val fireblocksOptions = FireblocksOptions.Builder()
.setLogLevel(Level.INFO)
.setEventHandler(object : FireblocksEventHandler {
override fun onEvent(event: Event) {
Timber.d("onEvent - $event")
fireEvent(event)
}
})
.setEnv(Environment.DEFAULT)
.build()
// initialize the SDK
val fireblocksSdk = Fireblocks.initialize(
context = applicationContext,
deviceId = deviceId);
messageHandler = fireblocksMessageHandler,
keyStorage = fireblocksKeyStorage,
fireblocksOptions = fireblocksOptions);
// Once initialized you can fetch it by deviceId
val fireblocksSdk = Fireblocks.getInstance(deviceId)
// Build your FireblocksOptions instance and pass an optional delegate that conforms to EventHandlerDelegate protocol
let fireblocksOptions = FireblocksOptions(env: .sandbox, eventHandlerDelegate: self)
// Generate an instance and pass two mandatory delegates that conform to MessageHandlerDelegate and KeyStorageDelegate
let instance = try Fireblocks.initialize(deviceId: deviceId, messageHandlerDelegate: self, keyStorageDelegate: keyStorageProvider, fireblocksOptions: fireblocksOptions)
// Once the SDK is initialized you can also get it by using the getInstance command
let instance = try Fireblocks.getInstance(deviceId: deviceId)
Updated 9 months ago