Initializing the SDK
Overall flow
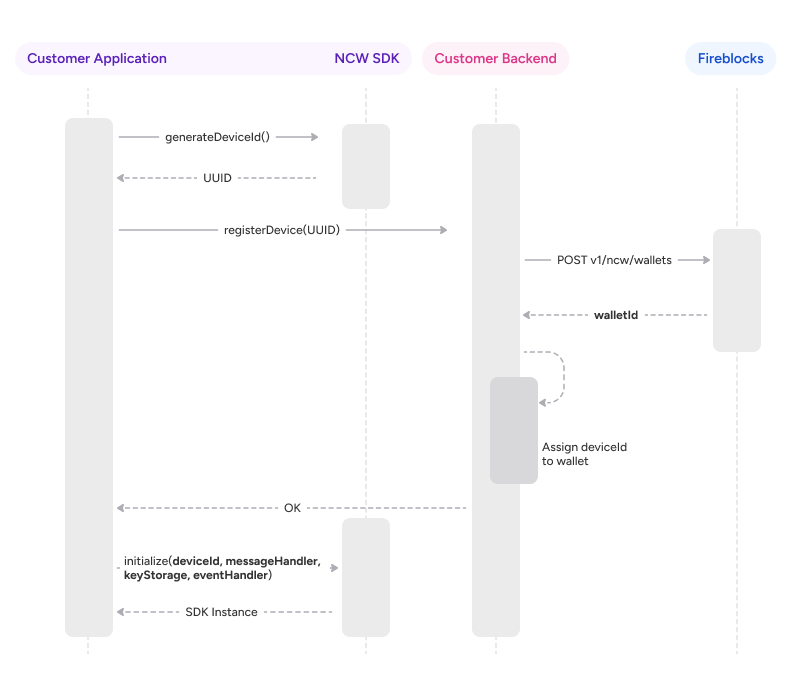
- Generate a device ID by calling the
generateDeviceId()
static method of the SDK. - Assign the
deviceId
to a wallet on your backend server. (POST /v1/wallets/
can be executed at this point.) - Initialize a new SDK instance.
Initialize the SDK
Generate a deviceId
. This value is unique per SDK instance.
Each non-custodial wallet (NCW) walletId
correlates to one or more deviceId
:
// generate a device Id, do it only once per SDK instance
const deviceId = FireblocksNCW.generateDeviceId()
// generate a device ID, do it only once per SDK instance
val deviceId = Fireblocks.generateDeviceId();
// generate a device Id, do it only once per SDK instance
let deviceId = Fireblocks.generateDeviceId()
Create an SDK instance
When creating a new SDK instance, provide the NCW instance with the following parameters:
deviceId
: The device ID associated with the end user and the Non-Custodial Wallet.messagesHandler
: The outgoing message handler.keyStorage
: The key storage handler.eventsHandler
(optional): The events handler.
Example
import { FireblocksNCW, IEventsHandler, IMessagesHandler, TEvent, InMemorySecureStorageProvider, FireblocksNCWFactory } from "@fireblocks/ncw-js-sdk";
// Initiate secure storage to hold generated data during SDK usage.
const secureStorageProvider = new InMemorySecureStorageProvider();
// Register a message handler to process outgoing message to your API
const messagesHandler: IMessagesHandler = {
handleOutgoingMessage: (message: string) => {
// Send the message to your API service
},
};
// Register an events handler to handle on various events that the SDK emitts
const eventsHandler: IEventsHandler = {
handleEvent: (event: TEvent) => {
if (event.type === 'key_descriptor_changed') {
// Do something when the event is fired.
}
},
};
const deviceId = generateDeviceId(); // UUID
let logger: IndexedDBLogger | null = await IndexedDBLoggerFactory({ deviceId, logger: ConsoleLoggerFactory() });
fireblocksNCW = await FireblocksNCWFactory({
env: ENV_CONFIG.NCW_SDK_ENV,
logLevel: "INFO",
deviceId,
messagesHandler,
eventsHandler,
secureStorageProvider,
logger,
});
// create your own implementation of the FireblocksMessageHandler
val fireblocksMessageHandler = FireblocksMessageHandlerImpl()
// create your own implementation of the FireblocksKeyStorage
val fireblocksKeyStorage = FireblocksKeyStorageImpl()
// build your FireblocksOptions instance
val fireblocksOptions = FireblocksOptions.Builder()
.setLogLevel(Level.INFO)
.setEventHandler(object : FireblocksEventHandler {
override fun onEvent(event: Event) {
Timber.d("onEvent - $event")
fireEvent(event)
}
})
.setEnv(Environment.DEFAULT)
.build()
// initialize the SDK
val fireblocksSdk = Fireblocks.initialize(
context = applicationContext,
deviceId = deviceId);
messageHandler = fireblocksMessageHandler,
keyStorage = fireblocksKeyStorage,
fireblocksOptions = fireblocksOptions);
// Once initialized you can fetch it by deviceId
val fireblocksSdk = Fireblocks.getInstance(deviceId)
// Build your FireblocksOptions instance and pass an optional delegate that conforms to EventHandlerDelegate protocol
let fireblocksOptions = FireblocksOptions(env: .sandbox, eventHandlerDelegate: self)
// Generate an instance and pass two mandatory delegates that conform to MessageHandlerDelegate and KeyStorageDelegate
let instance = try Fireblocks.initialize(deviceId: deviceId, messageHandlerDelegate: self, keyStorageDelegate: keyStorageProvider, fireblocksOptions: fireblocksOptions)
// Once the SDK is initialized you can also get it by using the getInstance command
let instance = try Fireblocks.getInstance(deviceId: deviceId)
Note
All SDKs (JS, Android, iOS) can be initialized with one of the following environments:
sandbox, production.The environment initialization value will affect the selection of the correct root certificate in the SDK. If your tenant resides in the production environment (whether it be testnet or mainnet), then choose production. If you are exploring NCW using the sandbox, then simply choose sandbox.
Additional deviceId information
A deviceId
is a UUID that you generate to reflect the identity of the client device. You should generate a deviceId
when you want a new device to join a wallet, such as during the end-user sign-up process.
When the NCW SDK initializes, it has no information about what wallet it belongs to. Your backend needs to associate the walletId
and deviceId
when RPC calls occur. Therefore, we recommend storing the associated walletId
and deviceId
in a way that is quick to fetch the walletId
by the deviceId
.
Please note that if a wallet with a deviceId
is fully initialized with keys on a physical device, you will only be able to transfer the keys that belong to that deviceId
to another device via the recovery procedure and that this procedure also renders the original device incapable of performing any MPC-related operations.
Updated about 1 year ago